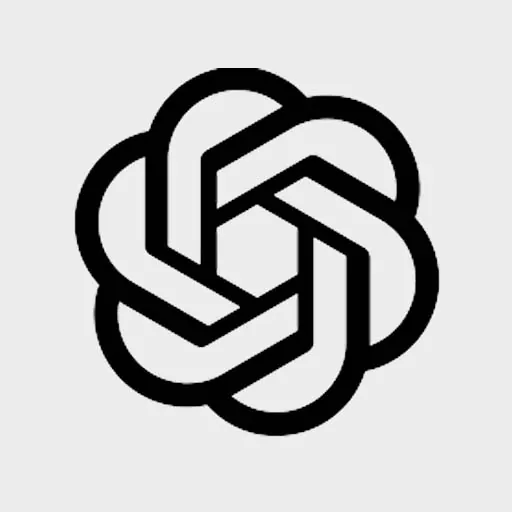
类型:人工智能
简介:一家开放人工智能研究和部署公司,推出了ChatGPT。
本教程将指导如何使用 OpenAI 的 DALL·E 模型生成或编辑图像。将涵盖图像生成、编辑和变体的基础知识,并提供实用的代码示例。目前,Images API 处于 Beta 版,默认速率限制为每分钟 50 张图像。
一、OpenAI图像生成概览
Images API 提供了三种与图像交互的方法:
- 基于文本提示生成图像
- 基于文本提示编辑现有图像
- 生成现有图像的变体
二、OpenAI图像生成
图像生成端点允许根据文本提示创建原始图像。生成的图像尺寸可以是 256×256、512×512 或 1024×1024 像素。可以使用 n 参数一次请求 1-10 张图像。
import openai response = openai.Image.create( prompt="一只白色暹罗猫", n=1, size="1024x1024" ) image_url = response['data'][0]['url']
提示:描述越详细,生成结果越符合预期。例如:
Prompt | Generation |
---|---|
一只白色暹罗猫 | 暹罗猫 |
一只好奇的白色暹罗猫,背光拍摄,摄影棚肖像 |
生成图像可通过 response_format 参数返回 URL 或 Base64 数据。URL 将在一小时后过期。
三、OpenAI图像编辑
图像编辑端点允许通过上传遮罩来编辑和扩展图像。遮罩的透明区域指示编辑范围,提示应描述完整的新图像,而不仅仅是擦除的部分。
response = openai.Image.create_edit( image=open("sunlit_lounge.png", "rb"), mask=open("mask.png", "rb"), prompt="一个阳光明媚的室内休息区,带有一个包含火烈鸟的泳池", n=1, size="1024x1024" ) image_url = response['data'][0]['url']
要求:上传的图像和遮罩必须是小于 4MB 的正方形 PNG 文件,且尺寸相同。
四、OpenAI图像变体
图像变体端点允许生成给定图像的变体。
response = openai.Image.create_variation( image=open("corgi_and_cat_paw.png", "rb"), n=1, size="1024x1024" ) image_url = response['data'][0]['url']
要求:输入图像必须是小于 4MB 的正方形 PNG 文件。
五、语言特定提示
1、Node.js 示例
使用内存中的图像数据:
// This is the Buffer object that contains your image data const buffer = [your image data]; // Set a `name` that ends with .png so that the API knows it's a PNG image buffer.name = "image.png"; const response = await openai.createImageVariation( buffer, 1, "1024x1024" );
2、TypeScript 示例
解决类型不匹配问题:
// Cast the ReadStream to `any` to appease the TypeScript compiler const response = await openai.createImageVariation( fs.createReadStream("image.png") as any, 1, "1024x1024" );
下面是一个类似的内存图像数据示例:
// This is the Buffer object that contains your image data const buffer: Buffer = [your image data]; // Cast the buffer to `any` so that we can set the `name` property const file: any = buffer; // Set a `name` that ends with .png so that the API knows it's a PNG image file.name = "image.png"; const response = await openai.createImageVariation( file, 1, "1024x1024" );
3、异常处理
API 请求可能因无效输入、速率限制等问题返回错误。使用 try…catch 语句处理错误:
try { const response = await openai.createImageVariation( fs.createReadStream("image.png"), 1, "1024x1024" ); console.log(response.data.data[0].url); } catch (error) { if (error.response) { console.log(error.response.status); console.log(error.response.data); } else { console.log(error.message); } }